Setup Svelte with Electron Forge for Desktop App Development 394 words.
Last Updated
The following guide demonstrates how to setup Svelte with Electron Forge. The end result is an electron app capable of hot reloading the render process.
💡 You can skip these steps by manually cloning the electron-forge-svelte template from github.
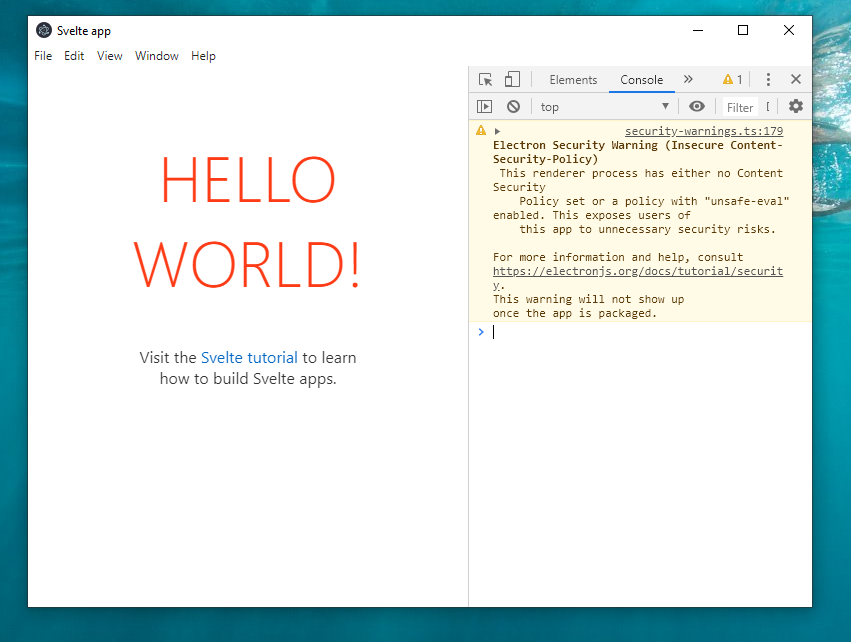
Svelte running with Electron Forge
Step 1 - Generate an Electron Forge App
First, generate an electron forge app.
npx create-electron-app my-app
cd my-app
Step 2 - Manually Integrate Svelte & Rollup
At this point, we need to manually add svelte to the project. This guide uses the official Svelte Template for the source code. Get ready for some surgical copy and pasting…
- Delete
index.html
andindex.css
from thesrc
directory. - Create a
public
folder, then copy theindex.html
,global.css
, andfavicon.png
from the Svelte Template. - Copy the
src/main.js
andsrc/App.svelte
files from the Svelte Template. To avoid confusion with Electron’s main process, change the name ofmain
tosvelte
. - Copy the
rollup.config.js
file from the svelte template.
Package.json Setup
Copy the dependencies and and devDependencies from the Svelte Template to your project’s package.json.
npm install
npm install concurrently
Copy the scripts from Svelte Template, the append svelte-
to each script name. Next, modify the start
script to concurrently run svelte and electron.
"scripts": {
"start": "concurrently \"npm:svelte-dev\" \"electron-forge start\"",
"package": "electron-forge package",
"make": "electron-forge make",
"publish": "electron-forge publish",
"lint": "echo \"No linting configured\"",
"svelte-build": "rollup -c",
"svelte-dev": "rollup -c -w",
"svelte-start": "sirv public"
},
Rollup Modification
Make the following changes to the rollup config file. Update the serve function to call svelte-start
instead of start
.
export default {
input: 'src/main.js', // <-- here
// ...omitted
}
//
function serve() {
// ...omitted
require('child_process').spawn('npm', ['run', 'svelte-start', '--', '--dev'], // <-- and here
HTML Modification
Electron resolves paths in your index.html
relative to the index.js
main process. Update your the html to use paths relative to the current working directory, i.e ./
.
<link rel="icon" type="image/png" href="./favicon.png" />
<link rel="stylesheet" href="./global.css" />
<link rel="stylesheet" href="./build/bundle.css" />
<script defer src="./build/bundle.js"></script>
Main Process Modification
Update the path to index.html.
mainWindow.loadFile(path.join(__dirname, '../public/index.html'));
Step 3 - Enable Live Reload
Enable live reloading with electron reload.
npm i electron-reload
Add the following line in your main process.
require('electron-reload')(__dirname, {
electron: path.join(__dirname, '../node_modules', '.bin', 'electron'),
awaitWriteFinish: true,
});
Expect a delay of approx 2s.
✔️ All done!